How to use the Python Console and the Editor inside CityEngine.
Python Console
Python scripts can be executed either in the Python console or from the Python Editor.
To open the Python console, display the Console (Window > Show Console), and select Python Console from the drop-down list below the small triangle in the toolbar.
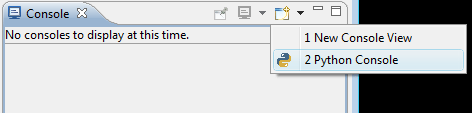
In the console, the specific CityEngine scripting commands as well as conventional Python commands can be typed. To execute a command line, press Enter. Use Ctrl + Space to show the command completion popup, which displays possible commands depending on your typing. The last used command can be called by pressing the up arrow key.
Note:
See Commands by category or Command reference for a list of available CityEngine methods.
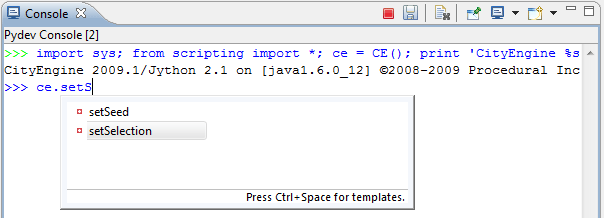
Python Editor
The Python Editor offers a more convenient way to edit and execute scripts. Create new script modules via File > New... > Python Module. Select the scripts folder of your current project as Source Folder, and specify a Name for your module.
Four module templates are available:
<Empty> | Creates an empty template |
Module: Class | Creates an empty python class |
Module: Export | Creates callback calls to be used with the Python-based exporter |
Module: Main | Creates an executable script |
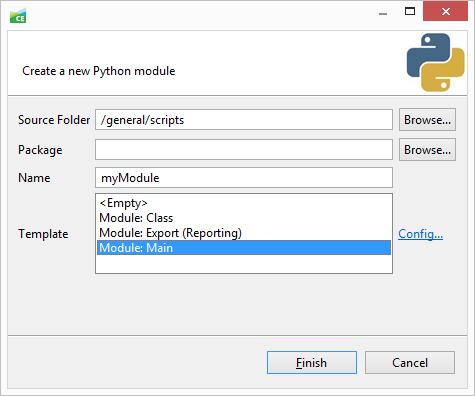
Choose the scripts folder of a CityEngine project as Source Folder, set a Name for the module and choose one of the templates.
New modules created via this dialog contain the following lines.
'''
Created on May 19, 2011
@author: andi
'''
from scripting import *
# get a CityEngine instance
ce = CE()
- A header
- an import statement that imports the CityEngine specific scripting module
- from this module, the main instance ce is created, which allows to calling all CityEngine Python commands
Running a script
To execute a script in the Editor, select Python > Run Script in the menu or press F9. (Focus needs to be on a Python script in the editor area to enable the Python menu).
Cancel all running Python scripts by choosing Cancel from the main toolbar (or by pressing Esc). As in the console, you can use Ctrl + Space to show the command completion.
Below you see the default parts of the Main template in black, and the added code in red. This script exports selected models as .obj files into the model folder of the current project.
'''
Created on May 19, 2011
@author: andi
'''
from scripting import *
# get a CityEngine instance
ce = CE()
def export(objects):
dir = ce.toFSPath("models/")
name = "pythonTriggeredExport"
settings = OBJExportModelSettings()
settings.setBaseName(name)
settings.setOutputPath(dir)
ce.export(objects, settings)
if __name__ == '__main__':
export(ce.selection())
pass
Importing modules
Just like in regular Python, additional modules can be imported.
>>> import random
>>> random.randint(0,100)
69
To load your custom scripts, make sure to add the script directory to the Python system path, before importing your module.
>>> import sys
>>> sys.path.append($PATH_TO_YOUR_SCRIPTS_DIRECTORY)
>>> import $YOUR_MODULE
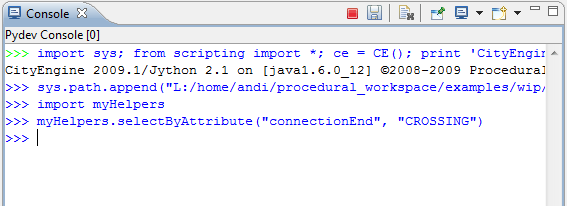
Scripts shortcut menu
To quickly start a Python script it can be placed in the scripts menu Scripts > Add scripts...
See also