How to import and export data using setting classes.
Import and export calls are configured using special settings classes, which define the type of importer or exporter to be used, as well as its settings. Available settings classes are listed on page Commands by category (Operations, Import, Export).
Import
In this example we want to import the shapefile testset_streets.shp, containing cartesian coordinates and not needing projection on import.
importSettings = OBJImportSettings()
a new instance of the OBJ import settings class is created, containing the default settings.
importSettings.setImportAsStaticModel(False)
Setting a property of the import settings class.
print importSettings.getOffset()
[0.0, 0.0, 0.0]
Just to make sure what the set defaults are, a property of the import settings class is printed.
objfile = ce.toFSPath("assets/sphere.obj")
ce.importFile(objfile, importSettings)
setting file to import, and triggering the import with the prepared settings..
ce.importFile(objfile, importSettings, True)
setting the interactive param to True will show the import dialog and wait for user input to continue. This can be helpful to check that import settings are set as expected.
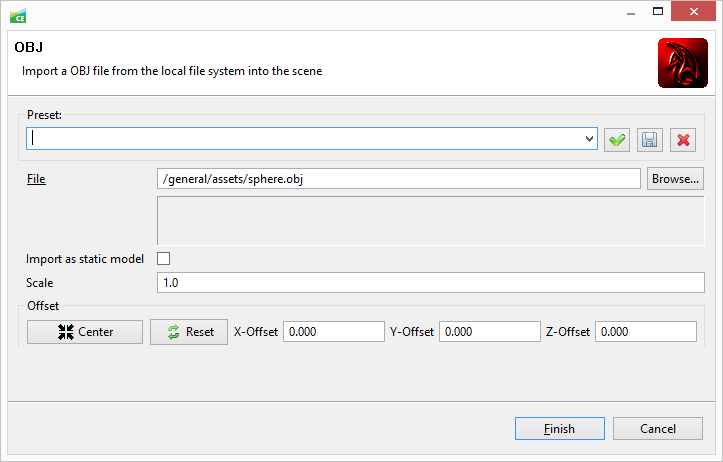
Export
In this example we want to export a selection of models as obj files, with file granularity set to one file per shape.
exportSettings = OBJExportModelSettings()
a new instance of the settings class for obj model export is created, containing the default settings.
exportSettings.setBaseName("testExport")
exportSettings.setOutputPath(ce.toFSPath("models/"))
exportSettings.setFileGranularity(OBJExportModelSettings.START_SHAPE)
We set specific properties of the settings class, such as name, path and the file granularity
Note:
String parameters for functions can either be set by using the predefined string constants of a class
exportSettings.setGranularityFile(OBJExportModelSettings.START_SHAPE
or by setting the string directly
exportSettings.setGranularityFile("START_SHAPE")
ce.export(ce.selection(), exportSettings)
Starting the actual export on selected objects, using the prepared settings class.
ce.export(ce.selection(), exportSettings, True)
setting the interactive param to True will show the import dialog and wait for user input to continue. This can be helpful to check that export settings are set as expected.
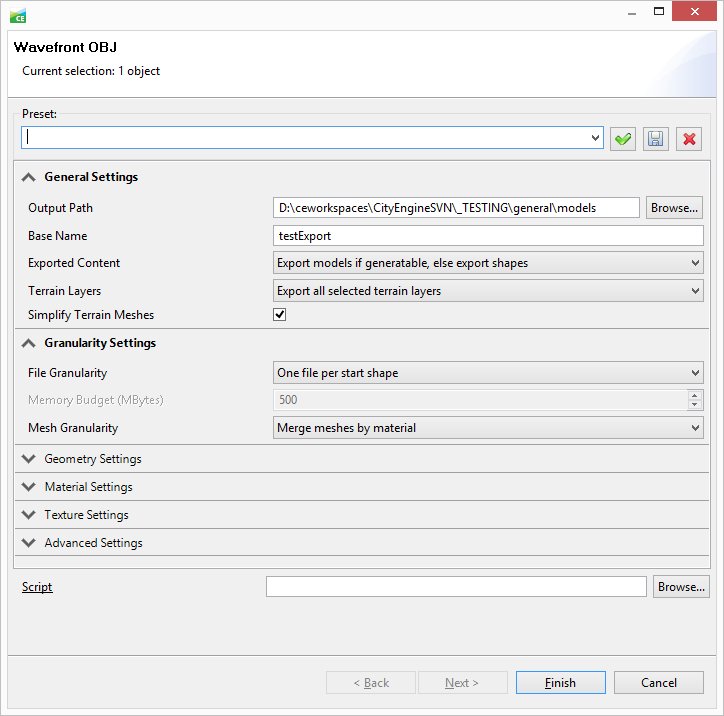
Cleanup Graph Operation
cleanupSettings = CleanupGraphSettings()
a new instance of the settings class for cleanup operation is created, containing the default settings.
cleanupSettings.setIntersectSegments(False)
cleanupSettings.setMergeNodes(False)
cleanupSettings.setSnapNodesToSegments(False)
cleanupSettings.setResolveConflictShapes(True)
We set specific properties of the settings class: All cleanup options except conflict resolution are disabled
ce.cleanupGraph(ce.selection(), cleanupSettings)
Starting the cleanup operation on selected objects, using the prepared settings class.
See also