Syntax
- string fileSearch(searchQuery)
Parameters
- searchQuery—stringSearch query to apply on list of all files in the workspace. See search queries.
Returns
An alphabetically sorted string list with all files in the workspace matching the searchQuery. Each entry is terminated with a ";" (semicolon).
Description
The fileSearch function lists all files in the workspace and matches their absolute path with the searchQuery. Search queries are relative to the current project (i.e. the project in which the current rule file resides) except if the searchQuery is based on an absolute workspace path (i.e. starts with a slash ("/") or a wildcard (* or ?). Pseudocode of the matching algorithm:
result = ""
for all open projects in workspace :
if project == current project:
result += all matching files in project, relative to assets folder
result += all matching files in project, relative to project folder
fi
result += all matching files in project, relative to workspace root
sort result
end
Search Queries
The CityEngine features advanced search queries supporting wildcards, regular expressions and file properties such as filetype. All files in the workspace are filtered with the query and the absolute workspace path is returned. Whitespace means AND.
Wildcards
The common wildcards characters '*' (asterisk character) and '?' (question mark) are supported. The asterisk substitutes for any zero or more characters, and the question mark substitutes for any one character.
Regular Expressions
Regular expressions allow for complex string patterns descriptions. A comprehensive introduction to regular expressions is out of scope for this manual, please refer to other sources such as Wikipedia or the specifiaction from the Open Group.
Regular expressions start with '$'. Always put the '$' as the FIRST character if your searchQuery is a regular expression. Note that with regular expressions, the semantics of the wildcards "*" and "?" changes. "*" matches the preceding element zero or more times, therefore use ".*" to emulate the "match anything" behaviour.
File Properties
The following properties can be querried:
- Name: Filename
- Ext: File extension
- Project: Project name
- Path: Filepath
Examples
In the following examples, we use a basic workspace with two projects and a few files. The examples are in rule.cga, i.e. MyProject is the current project.
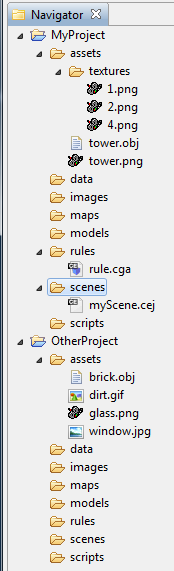
example.1: Wildcards
print(fileSearch("*.png"))
# result ="/MyProject/assets/textures/1.png;/MyProject/assets/textures/2.png;
/MyProject/assets/textures/4.png;/MyProject/assets/tower.png;
/OtherProject/assets/glass.png;"
// Because the searchQuery starts with an asterisk, //
// all projects are included in the search. //
example.2 Wildcards
print(fileSearch("?.png"))
# result = ";"
// There is no png file with a 1-character-name in the root directory or //
// the asset directory of the current project. //
example.3 Wildcards
print(fileSearch("*/?.png"))
# result = "/MyProject/assets/textures/1.png;/MyProject/assets/textures/2.png;
/MyProject/assets/textures/4.png;"
// Because of the leading asterisk, all folders are //
// searched for a png file with a 1-character-name. //
example.4 Wildcards
print(fileSearch("textures/*"))
# result = "/MyProject/assets/textures/1.png;
/MyProject/assets/textures/2.png;/MyProject/assets/textures/4.png;"
// All files in the "textures" subfolder of the asset //
// folder in the current project match. //
example.5 Regular Expression to select specific characters
print(fileSearch("$[12].png"))
# result = ";"
// There is no png file with name "1" or "2" in the root directory or //
// the asset directory of the current project. //
example.6 Regular Expression to select specific characters, part 2
print(fileSearch("$textures/[12].png"))
# result = "/MyProject/assets/textures/1.png;/MyProject/assets/textures/2.png;"
// All png files with name "1" or "2" in the "textures" subfolder of //
// the asset folder in the current project match. //
example.7 Regular Expression + Wildcard
print(fileSearch("$.*/[12].png"))
# result = "/MyProject/assets/textures/1.png;/MyProject/assets/textures/2.png;"
// All png files with name "1" or "2" match. //
// Note the period before the asterisk. //
example.8 Regular Expression to select a range of characters
print(fileSearch("$textures/[2-5].png"))
# result = "/MyProject/assets/textures/2.png;/MyProject/assets/textures/4.png;"
// All png files with name "2", "3, "4" or "5" //
// in the "textures" subfolder of the //
// asset folder in the current project match. //
example.9 Regular Expression + OR
print(fileSearch("$textures/[12].png|.*.obj"))
# result = /MyProject/assets/textures/1.png;/MyProject/assets/textures/2.png;
/MyProject/assets/tower.obj;/OtherProject/assets/brick.obj;
// All png files with name "1" or "2" in the //
// "textures" subfolder of the asset folder in the current //
project and all obj files in the workspace match. //
example.10 File Properties
print(fileSearch("Name=1.png"))
# result = "/MyProject/assets/textures/1.png;"
// Only one file matches the name excatly. //
example.11 File Properties, AND
print(fileSearch("Project=OtherProject Ext=obj"))
# result = "/OtherProject/assets/brick.obj;"
// Using white space, two or more queries can be anded. //
// Here the only file matching the project name and //
// the file extension is matched. //