Label | Explanation | Data Type |
Input Surface | The surface that will be used for interpolating z-values. | LAS Dataset Layer; Mosaic Layer; Raster Layer; Terrain Layer; TIN Layer; Image Service |
Input Features | The input features that will be processed. | Feature Layer |
Output Feature Class | The feature class that will be produced. | Feature Class |
Sampling Distance (Optional) | The spacing at which z-values will be interpolated. By default, this is a raster dataset's cell size or a triangulated surface's natural densification. | Double |
Z Factor (Optional) | The factor by which z-values will be multiplied. This is typically used to convert z linear units to match x,y linear units. The default is 1, which leaves elevation values unchanged. This parameter is not available if the spatial reference of the input surface has a z-datum with a specified linear unit. | Double |
Method (Optional) | Specifies the interpolation method that will be used to determine elevation values for the output features. The available options depend on the surface type.
| String |
Interpolate Vertices Only (Optional) | Specifies whether the interpolation will only occur along the vertices of an input feature, ignoring the sample distance option. When the input surface is a raster and the nearest neighbor interpolation method is selected, the z-values can only be interpolated at the feature vertices.
| Boolean |
Pyramid Level Resolution (Optional) | The z-tolerance or window-size resolution of the terrain pyramid level that will be used. The default is 0, or full resolution. | Double |
Preserve features partially outside surface
(Optional) | Specifies whether features with one or more vertices that fall outside the raster's data area will be retained in the output. This parameter is only available when the input surface is a raster and the nearest neighbor interpolation method is used.
| Boolean |
Available with 3D Analyst license.
Available with Spatial Analyst license.
Summary
Creates 3D features by interpolating z-values from a surface.
Illustration
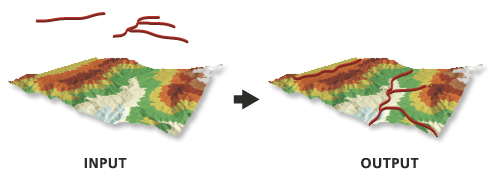
Usage
This tool creates 3D features using height values derived from overlapping portions of the input surface. A 3D polygon will only store z-values on its perimeter, since the interior of a 3D polygon will be randomly defined when it is rendered. For this reason, 3D polygons are generally not suitable for representing nonplanar height information. To generate a true representation of the surface, use the Interpolate Polygon To Multipatch tool.
Any curved line or polygon segments will be densified based on the Sampling Distance parameter value. If a sampling distance is not defined, this value will be derived from the input surface. For a raster, the default sampling size will be the raster's cell size. For a TIN, terrain, or LAS dataset, the default sampling will be based on the edges produced by the triangulated surface. If the curve is shorter than the sampling size, the curve will be simplified into a two-point line using its start and end points.
When using natural neighbors interpolation, consider specifying a sampling distance that's equal to or above half of the average point spacing of the data points in the surface.
When using the Interpolate Vertices Only parameter, features with vertices that fall outside the data area of the surface will not be part of the output unless the input surface is a raster and the nearest neighbor interpolation method is used.
Parameters
arcpy.ddd.InterpolateShape(in_surface, in_feature_class, out_feature_class, {sample_distance}, {z_factor}, {method}, {vertices_only}, {pyramid_level_resolution}, {preserve_features})
Name | Explanation | Data Type |
in_surface | The surface that will be used for interpolating z-values. | LAS Dataset Layer; Mosaic Layer; Raster Layer; Terrain Layer; TIN Layer; Image Service |
in_feature_class | The input features that will be processed. | Feature Layer |
out_feature_class | The feature class that will be produced. | Feature Class |
sample_distance (Optional) | The spacing at which z-values will be interpolated. By default, this is a raster dataset's cell size or a triangulated surface's natural densification. | Double |
z_factor (Optional) | The factor by which z-values will be multiplied. This is typically used to convert z linear units to match x,y linear units. The default is 1, which leaves elevation values unchanged. This parameter is not available if the spatial reference of the input surface has a z-datum with a specified linear unit. | Double |
method (Optional) | Specifies the interpolation method that will be used to determine elevation values for the output features. The available options depend on the surface type.
| String |
vertices_only (Optional) | Specifies whether the interpolation will only occur along the vertices of an input feature, ignoring the sample distance option.
| Boolean |
pyramid_level_resolution (Optional) | The z-tolerance or window-size resolution of the terrain pyramid level that will be used. The default is 0, or full resolution. | Double |
preserve_features (Optional) | Specifies whether features with one or more vertices that fall outside the raster's data area will be retained in the output. This parameter is only available when the input surface is a raster and the nearest neighbor interpolation method is used.
| Boolean |
Code sample
The following sample demonstrates the use of this tool in the Python window.
arcpy.env.workspace = "C:/data"
arcpy.ddd.InterpolateShape("my_tin", "roads.shp", "roads_interp.shp")
The following sample demonstrates the use of this tool in a stand-alone Python script.
'''*********************************************************************
Name: InterpolateShape Example
Description: This script demonstrates how to use InterpolateShape
on all 2D features in a target workspace.
*********************************************************************'''
# Import system modules
import arcpy
# Set local variables
inWorkspace = arcpy.GetParameterAsText(0)
surface = arcpy.GetParameterAsText(1)
try:
# Set default workspace
arcpy.env.workspace = inWorkspace
# Create list of feature classes in target workspace
fcList = arcpy.ListFeatureClasses()
if fcList:
for fc in fcList:
desc = arcpy.Describe(fc)
# Find 2D features
if not desc.hasZ:
# Set Local Variables
outFC = "{0}_3D.shp".format(desc.basename)
method = "BILINEAR"
# Execute InterpolateShape
arcpy.ddd.InterpolateShape(surface, fc, outFC,
10, 1, method, True)
else:
print("{0} is not a 2D feature.".format(fc))
else:
print("No feature classes were found in {0}.".format(env.workspace))
except arcpy.ExecuteError:
print(arcpy.GetMessages())
except Exception as err:
print(err)